Making a custom border shape in Flutter with ColorFiltered
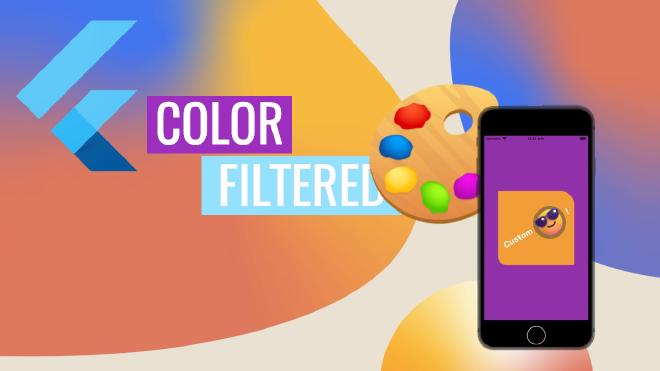
Table of Contents
Maybe a CustomPainter would be a better/easier solution, though
😉.I allways thought that creating a custom border shape in Flutter was a simple task, but I was wrong. I want to share with you the solution I found to achieve it.
Important note: I found this solution some time ago, and I’m not able to find the source of it. If you know the original author, please let me know so I can give him/her the credits!! 😄.
Why can’t we just use the border+borderradius properties? 🤔 #
This is the design my team wanted to achieve:
(This is just oversimplified version of it, but it’s enough to understand the problem)

Ok, this is not a super complex design, but I thought that it would be a simple task to achieve it. I mean, we just need to create a custom border shape, right?
That was my first thought when I started to work on this. I wanted to create a custom border shape for a widget, so I thought that I could just use the border and borderRadius properties of the BoxDecoration class.
return Container(
decoration: BoxDecoration(
border: Border(
top: BorderSide(
color: Colors.red.withOpacity(0.5),
width: 25,
),
bottom: BorderSide(
color: Colors.red.withOpacity(0.5),
width: 90,
),
left: BorderSide(
color: Colors.red.withOpacity(0.5),
width: 20,
),
right: BorderSide(
color: Colors.red.withOpacity(0.5),
width: 20,
),
),
),
);
So… this is what I got:
Not bad, let’s add some border radius to the container:
return Container(
// ...
borderRadius: const BorderRadius.all(Radius.circular(6)),
// ...
);
Let’s see the result
…A borderRadius can only be given for a uniform Border.
… oh, no! 😱

So it seems that we can’t use the border and borderRadius properties to achieve this design. 😞
ColorFiltered at the rescue! 😎 #
After some research, I found a solution that worked for me. I used the ColorFiltered widget to achieve it.
This widget allows us to apply a color filter to its child.
In this case, I used the BlendMode.srcOut mode to achieve the desired effect, with a Stack as a child, this Stack will have a DecoratedBox with a backgroundBlendMode of BlendMode.dstOut and a Container that will be the one that will determine the shape of the border.
return ColorFiltered(
colorFilter: ColorFilter.mode(
Colors.red.withOpacity(0.5),
BlendMode.srcOut,
),
child: Stack(
fit: StackFit.expand,
children: [
const DecoratedBox(
decoration: BoxDecoration(
color: Colors.black,
backgroundBlendMode: BlendMode.dstOut,
),
),
Container(
margin: const EdgeInsets.only(
top: 25,
bottom: 90,
left: 20,
right: 20,
),
decoration: const BoxDecoration(
color: Colors.black,
borderRadius: BorderRadius.all(Radius.circular(6)),
),
),
],
),
);
And this is the result:

Pretty cool, right? 😎
Conclusion 📝 #
In this post, I showed you how to use the ColorFiltered widget to create a custom border shape in Flutter.
Please, keep in mind that this is not the only way to achieve this! 😉
I hope you enjoyed it and that you found it useful.
If you have any questions or suggestions, feel free to leave a comment below. 😄
Thanks for reading! 🤓
The full source code for this post is available here 🔍